用户资料页
提示
此功能在 5.12.0 版本开始支持。
用户资料页展示了用户的基本信息。进入该页面,SDK 就会从数据库中获取用户信息显示。IMKit 提供基于 UIKit UITableView
的资料页类 RCProfileViewController
,资料页通过初始化传入不同的 viewModel 区分是当前用户资料页或者其他用户资料页。
IMKit 会话页面不会主动跳转用户资料页,开发者如果使用用户资料页,需要自行监听会话页面 RCConversationViewController
的事件(如头像点击 didTapCellPortrait:
方法)做跳转。
开通服务
使用此功能前,您须在控制台开通信息托管服务。
当前用户资料页
当前用户资料页包含标题、用户信息详情(头像、昵称、应用号、性别)。
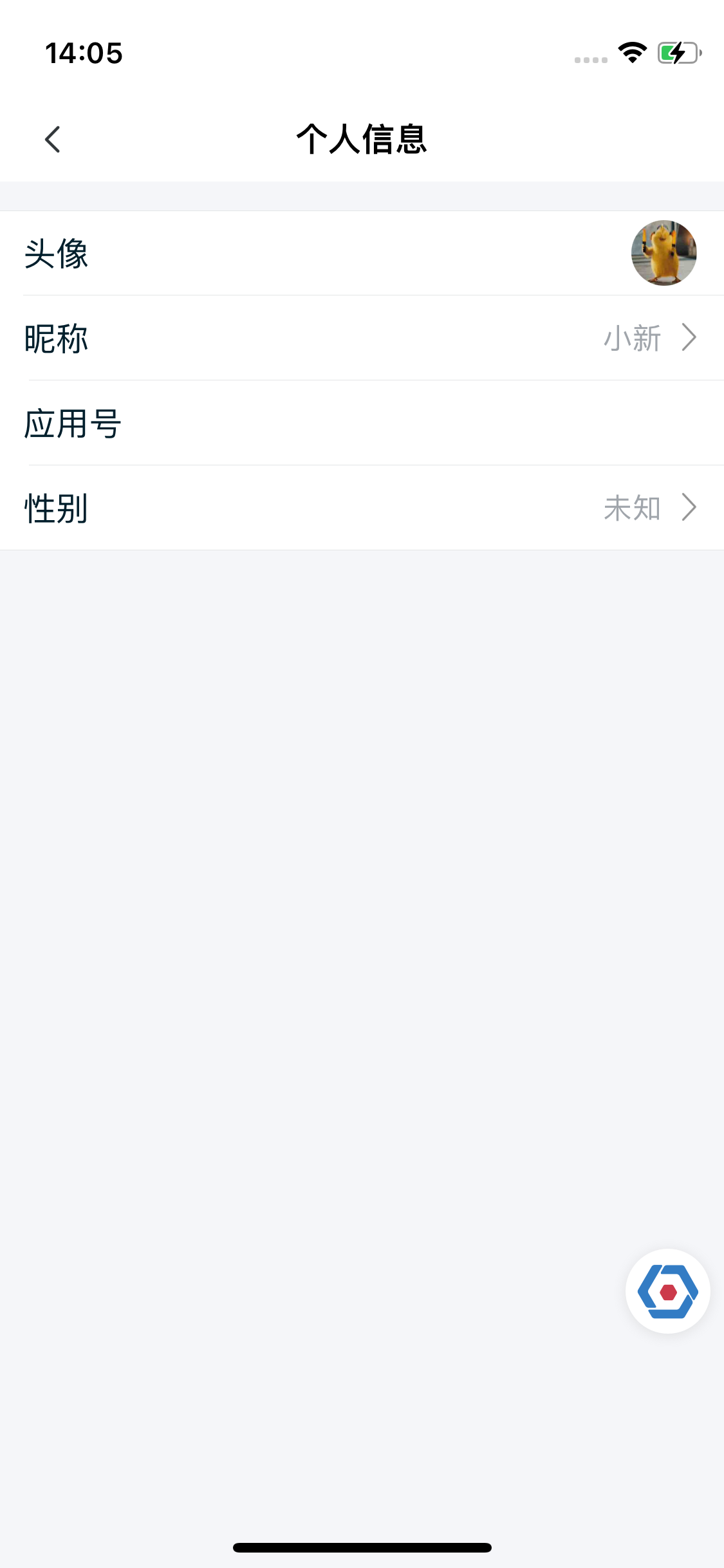
初始化
参数说明
参数 | 类型 | 说明 |
---|---|---|
viewModel | RCProfileViewModel | RCProfileViewController 的业务逻辑处理模块。 当前用户信息获取展示逻辑使用RCProfileViewModel 子类 RCMyProfileViewModel 初始化。 |
示例代码
Objective C
RCMyProfileViewModel *viewModel = [RCMyProfileViewModel new];
RCProfileViewController *vc = [[RCProfileViewController alloc] initWithViewModel:viewModel];
[self.navigationController pushViewController:vc animated:YES];
修改标题
用户资料页 RCProfileViewController
使用了系统的导航栏,默认有标题,可以在初始化后修改:
示例代码
Objective C
RCProfileViewController *vc = [[RCProfileViewController alloc] initWithViewModel:viewModel];
vc.title = @"custom title";
自定义当前用户资料页 cell
1. 自定义 RCCustomCell
Objective C
@interface RCCustomCell : UITableViewCell
@property (nonatomic, strong) UILabel *titleLabel;
@property (nonatomic, strong) UILabel *contentLabel;
@end
@implementation
// Cell 绘制
@end
2.自定义 RCProfileCustomCellViewModel, 继承 RCProfileCellViewModel
Objective C
@interface RCProfileCustomCellViewModel : RCProfileCellViewModel
@property (nonatomic, strong) NSString *title;
@property (nonatomic, strong) NSString *detail;
@end
@implementation RCProfileCustomCellViewModel
// 注册并自定义cell
- (UITableViewCell *)tableView:(UITableView *)tableView
cellForRowAtIndexPath:(NSIndexPath *)indexPath {
NSString *cellIdentifier = @"RCCustomCellIdentifier";
// 注册 cell
[tableView registerClass:RCCustomCell.class forCellReuseIdentifier:cellIdentifier];
// 返回 cell
RCCustomCell *cell = [tableView dequeueReusableCellWithIdentifier:cellIdentifier];
if (!cell) {
cell = [tableView dequeueReusableCellWithIdentifier:cellIdentifier];
}
//修改UI
cell.titleLabel.text = self.title;
cell.contentLabel.text = self.detail;
return cell;
}
// 返回 cell 高度
- (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath{
return 40;
}
@end
3. 设置添加 RCProfileViewModel 代理
在初始化资料页时设置 RCProfileViewModel
代理 RCProfileViewModelDelegate
:
Objective C
RCMyProfileViewModel *viewModel = [RCMyProfileViewModel new];
// 添加 代理
viewModel.delegate = self;
RCProfileViewController *vc = [[RCProfileViewController alloc] initWithViewModel:viewModel];